In this article, I am going to discuss how to create Custom HTML Helpers in ASP.NET MVC application. Please read our previous article, where we discussed how to Customizing Template Helpers in ASP.NET MVC application. As part of this article, we are going to discuss the following two things
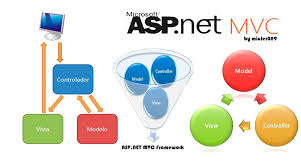
- How we can display Images in an ASP.NET MVC application?
- How to create Custom HTML Helpers in MVC to display images?
As we already discussed the HTML helper is a method that returns an HTML string. Then this HTML string is rendered in a view. ASP.NET MVC provides many built-in HTML helper methods that we can directly use in a view. The MVC framework also provides the facility to create Custom HTML Helpers in ASP.NET MVC application. Once you create your custom HTML helper method then you can reuse it many times.
Lets understand Custom HTML Helpers in MVC with an example.
In this demo, we are going to display the employee details along with the Employee photo as shown in the below image.
Creating an empty ASP.NET MVC application
First, create an empty ASP.NET MVC application with the name CustomHTMLHelper. Then create one model class with the name Employee within the Models Folder and then copy and paste the following code in it.
Then add a folder with the Name Photos to the project.
To do this, Right-click on the Project and select Add Folder and then rename the folder as “Photos“. Then download and add the following image within the Photos Folder. Rename the image name as MyPhoto.png.
Creating Controller:
Now add a controller with the name EmployeeController within the Controllers folder and then copy and paste the below codes.
Adding View:
Add the Details view and then copy and paste the following codes in it.
Now Run the application and navigates to the URL http://localhost:61629/Employee/Details It will produce the following output.
Notice instead of rendering the photo, the PhotoPath and AlternateText property values are displayed.
How to display an image in ASP.NET MVC Application?
Replace the above code with the following code.
Notice that, now we are using the Url.Content() HTML helper method. This method resolves a URL for a resource when we pass it the relative path. Now, run the application, and notice that the image is displayed as expected as shown in the below image.
We use the below code to render Image in ASP.NET MVC application. We are building the image tag, by passing the values for “src†and “alt†attributes.
<img src=â€@Url.Content(@Model.Photo)†alt=â€@Model.AlternateText†/>
Though the above code is not very complex, it still makes sense to move this logic into its own helper method. We dont want any complicated logic in our views. Views should be as simple as possible. Dont you think, it would be very nice, if we can render the image, using Image() HTML helper method as shown below.
@Html.Image(Model.Photo, Model.AlternateText)
But, ASP.NET MVC does not provide any built-in Image() HTML helper. So, lets build our own custom image HTML helper method. Lets take a step back and understand HTML helper methods. The HTML helper method simply returns a string. To generate a textbox, we use the following code in our view.
@Html.TextBox(“TextBox Nameâ€)
So, here TextBox() is an extension method defined in HtmlHelper class. In the above code, Html is the property of the View, which returns an instance of the HtmlHelper class.
Creating Image() extension method, to HtmlHelper class.
Right-click on the project and add the “CustomHelpers†folder. Then Right-click on the “CustomHelpers†folder and add the “CustomHelpers.cs†class file. Copy and paste the following code in it. The code is commented and self-explanatory. TagBuilder class is in System.Web.Mvc namespace.
To use the custom Image() HTML helper in Details.cshtml view, please include the following using statement in Details.cshtml
@using CustomHTMLHelper.CustomHelpers;
As we intend to use this Image() HTML helper, in all our views, lets include “CustomHTMLHelper.CustomHelpers†namespace in web.config that is present in the Views Folder. This eliminates the need to include the namespace, in each and every view.
Now use the following code to display an Image
@Html.Image(Model.Photo, Model.AlternateText)
If you intend to use the Image() custom HTML helper, only with a set of views, then, include a web.config file in the specific views folder, and then specify the namespace in it.